PHP Interview Questions And Answers
1) What is the difference between PHP running under the Apache module and running under CGI program?
Ans: When PHP is running under the apache module the php.ini settings will be loaded into memory when we start the Apache web server. Whereas when running under CGI mode for each and every request the settings will be loaded into memory. If you made any change in php.ini and if you are running under Apache, you must restart it.
2) What are the types of XML parsers available in PHP? Ans: There are two types of XML parsers available which is “Tree” based parser and “Event” based parser. “Tree” based parsers are very useful when we parse a small XML files or strings, an ideal example would be a SimpleXML library in PHP. “Event” based parsers are very useful to parse large XML files, the example would be SAX parser in PHP.
3) How do you achieve polymorphism in PHP? Ans: Using the magic methods: set(), get(), isset()
4) How do you achieve multiple inheritances in PHP? Ans: Using Interface
5) What are the characters will be automatically escaped when you enable “magic_quotes_gpc” in your php.ini file? Ans: Single quote (‘), Double quote (“) and null (NULL)
6) Name few functions in PHP to execute OS commands? Ans: system, exec, passthrough etc.
7) How do you achieve Multi-Threading in PHP? Ans: Using pcntl forking, which will be used in UNIX like OS in CLI mode.
8) What’s PHP ? Ans: The PHP Hypertext Preprocessor is a programming language that allows web developers to create dynamic content that interacts with databases. PHP is basically used for developing web based software applications.
9) What Is a Session? Ans: A session is a logical object created by the PHP engine to allow you to preserve data across subsequent HTTP requests. There is only one session object available to your PHP scripts at any time. Data saved to the session by a script can be retrieved by the same script or another script when requested from the same visitor. Sessions are commonly used to store temporary data to allow multiple PHP pages to offer a complete functional transaction for the same visitor.
10) What is meant by PEAR in php? Ans: PEAR is short for “PHP Extension and Application Repository” and is pronounced just like the fruit. The purpose of PEAR is to provide: A structured library of open-sourced code for PHP users A system for code distribution and package maintenance A standard style for code written in PHP The PHP Foundation Classes (PFC), The PHP Extension Community Library (PECL), A web site, mailing lists and download mirrors to support the PHP/PEAR community PEAR is a community-driven project with the PEAR Group as the governing body. The project has been founded by Stig S. Bakken in 1999 and quite a lot of people have joined the project since then.
11) How can we know the number of days between two given dates using PHP? Ans: Simple arithmetic: $date1 = date(‘Y-m-d’); $date2 = ‘2006-07-01’; $days = (strtotime() – strtotime()) / (60 * 60 * 24); echo “Number of days since ‘2006-07-01’: $days”;
12) How can we repair a MySQL table? Ans: The syntex for repairing a mysql table is: REPAIR TABLE tablename REPAIR TABLE tablename QUICK REPAIR TABLE tablename EXTENDED This command will repair the table specified. If QUICK is given, MySQL will do a repair of only the index tree. If EXTENDED is given, it will create index row by row.
13) What is the difference between $message and $$message? Ans: $message is a simple variable whereas $$message is a reference variable. Example: $user = ‘bob’ is equivalent to $holder = ‘user’; $$holder = ‘bob’;
14) What Is a Persistent Cookie? Ans: A persistent cookie is a cookie which is stored in a cookie file permanently on the browser’s computer. By default, cookies are created as temporary cookies which stored only in the browser’s memory. When the browser is closed, temporary cookies will be erased. You should decide when to use temporary cookies and when to use persistent cookies based on their differences: *Temporary cookies can not be used for tracking long-term information. *Persistent cookies can be used for tracking long-term information. *Temporary cookies are safer because no programs other than the browser can access them. *Persistent cookies are less secure because users can open cookie files see the cookie values.
15) What does a special set of tags do in PHP? Ans: The output is displayed directly to the browser.
16) What are the differences between DROP a table and TRUNCATE a table? Ans: DROP TABLE table_name – This will delete the table and its data. TRUNCATE TABLE table_name – This will delete the data of the table, but not the table definition.
17) How do you call a constructor for a parent class? Ans: parent::constructor($value)
18) What are the different types of errors in php? Ans: Here are three basic types of runtime errors in PHP: 1. Notices: These are trivial, non-critical errors that PHP encounters while executing a script – for example, accessing a variable that has not yet been defined. By default, such errors are not displayed to the user at all – although you can change this default behavior. 2. Warnings: These are more serious errors – for example, attempting to include() a file which does not exist. By default, these errors are displayed to the user, but they do not result in script termination. 3. Fatal errors: These are critical errors – for example, instantiating an object of a non-existent class, or calling a non-existent function. These errors cause the immediate termination of the script, and PHP’s default behavior is to display them to the user when they take place. Internally, these variations are represented by twelve different error types
19) What’s the special meaning of _sleep and _wakeup? Ans: _sleep returns the array of all the variables than need to be saved, while _wakeup retrieves them.
20) What are the MySQL database files stored in system ? Ans: Data is stored in name.myd Table structure is stored in name.frm Index is stored in name.myi
21) How can we register the variables into a session? Ans: session_register($session_var); $_SESSION[‘var’] = ‘value’;
22) What is the difference between characters \023 and \x23? Ans: The first one is octal 23, the second is hex 23.
23) With a heredoc syntax, do I get variable substitution inside the heredoc contents? Ans: Yes.
24) :What is meant by MIME? Ans: MIME is Multipurpose Internet Mail Extensions is an Internet standard for the format of e-mail. However browsers also uses MIME standard to transmit files. MIME has a header which is added to a beginning of the data. When browser sees such header it shows the data as it would be a file (for example image) Some examples of MIME types: audio/x-ms-wmp image/png application/x-shockwave-flash
25) How can we create a database using PHP and mysql? Ans: We can create MySQL database with the use of mysql_create_db($databaseName) to create a database.
26) How many ways we can retrieve the date in result set of mysql using php? Ans: As individual objects so single record or as a set or arrays.
27) What is the difference between PHP4 and PHP5? Ans: PHP4 cannot support oops concepts and Zend engine 1 is used. PHP5 supports oops concepts and Zend engine 2 is used. Error supporting is increased in PHP5. XML and SQLLite will is increased in PHP5.
28) How do I accomplish that with PHP? Ans: On large strings that need to be formatted according to some length specifications, use wordwrap() or chunk_split().
29) What’s the difference between htmlentities() and htmlspecialchars()? Ans: htmlspecialchars only takes care of <, >, single quote ‘, double quote ” and ampersand. htmlentities translates all occurrences of character sequences that have different meaning in HTML.
30) How can we extract string “abc.com” from a string “mailto:[email protected]?subject=Feedback” using regular expression of PHP? Ans: $text = “mailto:[email protected]?subject=Feedback”; preg_match(‘|.*@([^?]*)|’, $text, $output); echo $output[1]; Note that the second index of $output, $output[1], gives the match, not the first one, $output[0]. So if md5() generates the most secure hash, why would you ever use the less secure
31) crc32() and sha1()? Ans: Crypto usage in PHP is simple, but that doesn’t mean it’s free. First off, depending on the data that you’re encrypting, you might have reasons to store a 32-bit value in the database instead of the 160-bit value to save on space. Second, the more secure the crypto is, the longer is the computation time to deliver the hash value. A high volume site might be significantly slowed down, if frequent md5() generation is required.
32) How can we destroy the session, how can we unset the variable of a session? Ans: session_unregister() – Unregister a global variable from the current session session_unset() – Free all session variables
33) What are the different functions in sorting an array? Ans: Sorting functions in PHP: asort() arsort() ksort() krsort() uksort() sort() natsort() rsort()
34) How can we know the count/number of elements of an array? Ans: 2 ways: a) sizeof($array) – This function is an alias of count() b) count($urarray) – This function returns the number of elements in an array. Interestingly if you just pass a simple var instead of an array, count() will return 1.
35) How many ways we can pass the variable through the navigation between the pages? Ans: At least 3 ways: 1. Put the variable into session in the first page, and get it back from session in the next page. 2. Put the variable into cookie in the first page, and get it back from the cookie in the next page. 3. Put the variable into a hidden form field, and get it back from the form in the next page.
36) What is the maximum length of a table name, a database name, or a field name in MySQL? Ans: Database name: 64 characters Table name: 64 characters Column name: 64 characters
37) How many values can the SET function of MySQL take? Ans: MySQL SET function can take zero or more values, but at the maximum it can take 64 values.
38) What are the other commands to know the structure of a table using MySQL commands except EXPLAIN command? Ans: DESCRIBE table_name;
39) How can we find the number of rows in a table using MySQL? Ans: Use this for MySQL SELECT COUNT(*) FROM table_name;
40) What’s the difference between md5(), crc32() and sha1() crypto on PHP? Ans: The major difference is the length of the hash generated. CRC32 is, evidently, 32 bits, while sha1() returns a 128 bit value, and md5() returns a 160 bit value. This is important when avoiding collisions.
41) How can we find the number of rows in a result set using PHP? Ans: Here is how can you find the number of rows in a result set in PHP: $result = mysql_query($any_valid_sql, $database_link); $num_rows = mysql_num_rows($result); echo “$num_rows rows found”;
42) How many ways we can we find the current date using MySQL? Ans: SELECT CURDATE(); SELECT CURRENT_DATE(); SELECT CURTIME(); SELECT CURRENT_TIME();
42) Give the syntax of GRANT commands? Ans: The generic syntax for GRANT is as following GRANT [rights] on [database] TO [username@hostname] IDENTIFIED BY [password] Now rights can be: a) ALL privileges b) Combination of CREATE, DROP, SELECT, INSERT, UPDATE and DELETE etc. We can grant rights on all database by using h *.* or some specific database by database.* or a specific table by database.table_name.
43) Give the syntax of REVOKE commands? Ans: The generic syntax for revoke is as following REVOKE [rights] on [database] FROM [username@hostname] Now rights can be: a) ALL privileges b) Combination of CREATE, DROP, SELECT, INSERT, UPDATE and DELETE etc. We can grant rights on all database by using *.* or some specific database by database.* or a specific table by database.table_name.
2) What are the types of XML parsers available in PHP? Ans: There are two types of XML parsers available which is “Tree” based parser and “Event” based parser. “Tree” based parsers are very useful when we parse a small XML files or strings, an ideal example would be a SimpleXML library in PHP. “Event” based parsers are very useful to parse large XML files, the example would be SAX parser in PHP.
3) How do you achieve polymorphism in PHP? Ans: Using the magic methods: set(), get(), isset()
4) How do you achieve multiple inheritances in PHP? Ans: Using Interface
5) What are the characters will be automatically escaped when you enable “magic_quotes_gpc” in your php.ini file? Ans: Single quote (‘), Double quote (“) and null (NULL)
6) Name few functions in PHP to execute OS commands? Ans: system, exec, passthrough etc.
7) How do you achieve Multi-Threading in PHP? Ans: Using pcntl forking, which will be used in UNIX like OS in CLI mode.
8) What’s PHP ? Ans: The PHP Hypertext Preprocessor is a programming language that allows web developers to create dynamic content that interacts with databases. PHP is basically used for developing web based software applications.
9) What Is a Session? Ans: A session is a logical object created by the PHP engine to allow you to preserve data across subsequent HTTP requests. There is only one session object available to your PHP scripts at any time. Data saved to the session by a script can be retrieved by the same script or another script when requested from the same visitor. Sessions are commonly used to store temporary data to allow multiple PHP pages to offer a complete functional transaction for the same visitor.
10) What is meant by PEAR in php? Ans: PEAR is short for “PHP Extension and Application Repository” and is pronounced just like the fruit. The purpose of PEAR is to provide: A structured library of open-sourced code for PHP users A system for code distribution and package maintenance A standard style for code written in PHP The PHP Foundation Classes (PFC), The PHP Extension Community Library (PECL), A web site, mailing lists and download mirrors to support the PHP/PEAR community PEAR is a community-driven project with the PEAR Group as the governing body. The project has been founded by Stig S. Bakken in 1999 and quite a lot of people have joined the project since then.
11) How can we know the number of days between two given dates using PHP? Ans: Simple arithmetic: $date1 = date(‘Y-m-d’); $date2 = ‘2006-07-01’; $days = (strtotime() – strtotime()) / (60 * 60 * 24); echo “Number of days since ‘2006-07-01’: $days”;
12) How can we repair a MySQL table? Ans: The syntex for repairing a mysql table is: REPAIR TABLE tablename REPAIR TABLE tablename QUICK REPAIR TABLE tablename EXTENDED This command will repair the table specified. If QUICK is given, MySQL will do a repair of only the index tree. If EXTENDED is given, it will create index row by row.
13) What is the difference between $message and $$message? Ans: $message is a simple variable whereas $$message is a reference variable. Example: $user = ‘bob’ is equivalent to $holder = ‘user’; $$holder = ‘bob’;
14) What Is a Persistent Cookie? Ans: A persistent cookie is a cookie which is stored in a cookie file permanently on the browser’s computer. By default, cookies are created as temporary cookies which stored only in the browser’s memory. When the browser is closed, temporary cookies will be erased. You should decide when to use temporary cookies and when to use persistent cookies based on their differences: *Temporary cookies can not be used for tracking long-term information. *Persistent cookies can be used for tracking long-term information. *Temporary cookies are safer because no programs other than the browser can access them. *Persistent cookies are less secure because users can open cookie files see the cookie values.
15) What does a special set of tags do in PHP? Ans: The output is displayed directly to the browser.
16) What are the differences between DROP a table and TRUNCATE a table? Ans: DROP TABLE table_name – This will delete the table and its data. TRUNCATE TABLE table_name – This will delete the data of the table, but not the table definition.
17) How do you call a constructor for a parent class? Ans: parent::constructor($value)
18) What are the different types of errors in php? Ans: Here are three basic types of runtime errors in PHP: 1. Notices: These are trivial, non-critical errors that PHP encounters while executing a script – for example, accessing a variable that has not yet been defined. By default, such errors are not displayed to the user at all – although you can change this default behavior. 2. Warnings: These are more serious errors – for example, attempting to include() a file which does not exist. By default, these errors are displayed to the user, but they do not result in script termination. 3. Fatal errors: These are critical errors – for example, instantiating an object of a non-existent class, or calling a non-existent function. These errors cause the immediate termination of the script, and PHP’s default behavior is to display them to the user when they take place. Internally, these variations are represented by twelve different error types
19) What’s the special meaning of _sleep and _wakeup? Ans: _sleep returns the array of all the variables than need to be saved, while _wakeup retrieves them.
20) What are the MySQL database files stored in system ? Ans: Data is stored in name.myd Table structure is stored in name.frm Index is stored in name.myi
21) How can we register the variables into a session? Ans: session_register($session_var); $_SESSION[‘var’] = ‘value’;
22) What is the difference between characters \023 and \x23? Ans: The first one is octal 23, the second is hex 23.
23) With a heredoc syntax, do I get variable substitution inside the heredoc contents? Ans: Yes.
24) :What is meant by MIME? Ans: MIME is Multipurpose Internet Mail Extensions is an Internet standard for the format of e-mail. However browsers also uses MIME standard to transmit files. MIME has a header which is added to a beginning of the data. When browser sees such header it shows the data as it would be a file (for example image) Some examples of MIME types: audio/x-ms-wmp image/png application/x-shockwave-flash
25) How can we create a database using PHP and mysql? Ans: We can create MySQL database with the use of mysql_create_db($databaseName) to create a database.
26) How many ways we can retrieve the date in result set of mysql using php? Ans: As individual objects so single record or as a set or arrays.
27) What is the difference between PHP4 and PHP5? Ans: PHP4 cannot support oops concepts and Zend engine 1 is used. PHP5 supports oops concepts and Zend engine 2 is used. Error supporting is increased in PHP5. XML and SQLLite will is increased in PHP5.
28) How do I accomplish that with PHP? Ans: On large strings that need to be formatted according to some length specifications, use wordwrap() or chunk_split().
29) What’s the difference between htmlentities() and htmlspecialchars()? Ans: htmlspecialchars only takes care of <, >, single quote ‘, double quote ” and ampersand. htmlentities translates all occurrences of character sequences that have different meaning in HTML.
30) How can we extract string “abc.com” from a string “mailto:[email protected]?subject=Feedback” using regular expression of PHP? Ans: $text = “mailto:[email protected]?subject=Feedback”; preg_match(‘|.*@([^?]*)|’, $text, $output); echo $output[1]; Note that the second index of $output, $output[1], gives the match, not the first one, $output[0]. So if md5() generates the most secure hash, why would you ever use the less secure
31) crc32() and sha1()? Ans: Crypto usage in PHP is simple, but that doesn’t mean it’s free. First off, depending on the data that you’re encrypting, you might have reasons to store a 32-bit value in the database instead of the 160-bit value to save on space. Second, the more secure the crypto is, the longer is the computation time to deliver the hash value. A high volume site might be significantly slowed down, if frequent md5() generation is required.
32) How can we destroy the session, how can we unset the variable of a session? Ans: session_unregister() – Unregister a global variable from the current session session_unset() – Free all session variables
33) What are the different functions in sorting an array? Ans: Sorting functions in PHP: asort() arsort() ksort() krsort() uksort() sort() natsort() rsort()
34) How can we know the count/number of elements of an array? Ans: 2 ways: a) sizeof($array) – This function is an alias of count() b) count($urarray) – This function returns the number of elements in an array. Interestingly if you just pass a simple var instead of an array, count() will return 1.
35) How many ways we can pass the variable through the navigation between the pages? Ans: At least 3 ways: 1. Put the variable into session in the first page, and get it back from session in the next page. 2. Put the variable into cookie in the first page, and get it back from the cookie in the next page. 3. Put the variable into a hidden form field, and get it back from the form in the next page.
36) What is the maximum length of a table name, a database name, or a field name in MySQL? Ans: Database name: 64 characters Table name: 64 characters Column name: 64 characters
37) How many values can the SET function of MySQL take? Ans: MySQL SET function can take zero or more values, but at the maximum it can take 64 values.
38) What are the other commands to know the structure of a table using MySQL commands except EXPLAIN command? Ans: DESCRIBE table_name;
39) How can we find the number of rows in a table using MySQL? Ans: Use this for MySQL SELECT COUNT(*) FROM table_name;
40) What’s the difference between md5(), crc32() and sha1() crypto on PHP? Ans: The major difference is the length of the hash generated. CRC32 is, evidently, 32 bits, while sha1() returns a 128 bit value, and md5() returns a 160 bit value. This is important when avoiding collisions.
41) How can we find the number of rows in a result set using PHP? Ans: Here is how can you find the number of rows in a result set in PHP: $result = mysql_query($any_valid_sql, $database_link); $num_rows = mysql_num_rows($result); echo “$num_rows rows found”;
42) How many ways we can we find the current date using MySQL? Ans: SELECT CURDATE(); SELECT CURRENT_DATE(); SELECT CURTIME(); SELECT CURRENT_TIME();
42) Give the syntax of GRANT commands? Ans: The generic syntax for GRANT is as following GRANT [rights] on [database] TO [username@hostname] IDENTIFIED BY [password] Now rights can be: a) ALL privileges b) Combination of CREATE, DROP, SELECT, INSERT, UPDATE and DELETE etc. We can grant rights on all database by using h *.* or some specific database by database.* or a specific table by database.table_name.
43) Give the syntax of REVOKE commands? Ans: The generic syntax for revoke is as following REVOKE [rights] on [database] FROM [username@hostname] Now rights can be: a) ALL privileges b) Combination of CREATE, DROP, SELECT, INSERT, UPDATE and DELETE etc. We can grant rights on all database by using *.* or some specific database by database.* or a specific table by database.table_name.
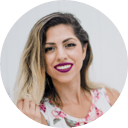
Chandanakatta
Author
Hey there! I shoot some hoops when I’m not drowned in the books, sitting by the side of brooks.